Splash Screen Loading Animation
A splash screen usually appears while a app or game launching. Splash Screen is not much important for an app. It increases the app launching time. Most of the applications are using splash screen. Gaming app mostly needs the splash screen. But some poor developers implements splash screen as an activity for an app with some delays. Splash Screen must contains minimum no.of layouts. Then only it launches quickly.
In this tutorial I simply used an AnimationSet for an View to implement loading animation.
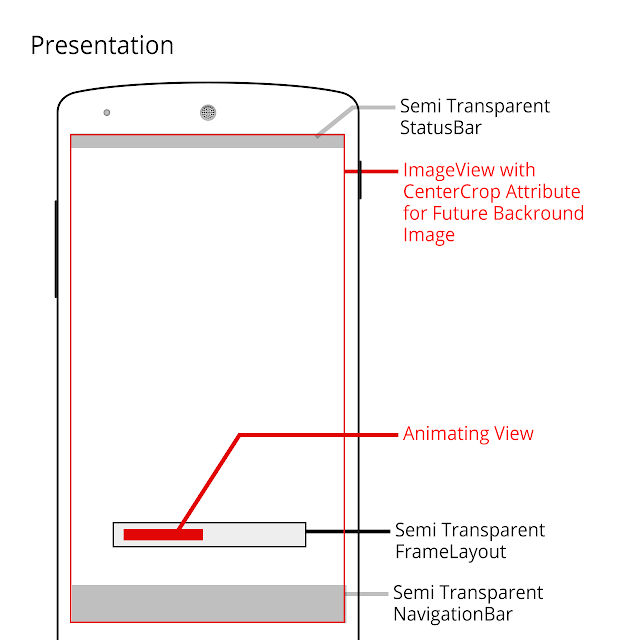
1. Start with creating new android Studio Project with an empty Activity.
2. Make sure your activity extends with Activity. Don’t use AppCompactActivity. Because The Theme We are gonna be use requires does not support AppCompactActivity.
3. Edit your “styles.xml” file.
styles.xml <resources>
<style name="AppTheme" parent="android:Theme.Holo.NoActionBar.TranslucentDecor">
<item name="android:windowBackground">@android:color/transparent</item>
<item name="android:windowNoTitle">true</item>
</style>
</resources>
slideout.xml<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
android:fillAfter="true">
<scale
android:duration="500"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:startOffset="600"
android:toXScale="0.0"
android:toYScale="1.0" />
</set>
5. Import necessary future drawable.future_image.png6. Edit your “activity_main.xml”.
activity_main.xml<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"
android:src="@drawable/future_image" />
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="100dp"
android:layout_marginLeft="125px"
android:layout_marginRight="125px"
android:background="#55ffffff"
android:padding="5dp">
<View
android:id="@+id/view_progress"
android:layout_width="250px"
android:layout_height="20dp"
android:layout_gravity="center"
android:background="@color/colorPrimaryDark" />
</FrameLayout>
</RelativeLayout>
7. Implement animation in your "MainActivity.java". MainActivity.javapackage info.androidramp.splashsample;
/**
* Created by Thanvandh on 27/12/2015.
*/
import android.app.Activity;
import android.content.res.Resources;
import android.os.Bundle;
import android.view.View;
import android.view.animation.AnimationSet;
import android.view.animation.AnimationUtils;
import android.view.animation.ScaleAnimation;
import android.view.animation.TranslateAnimation;
public class MainActivity extends Activity {
View viewProgress;
AnimationSet animationSet;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
viewProgress = findViewById(R.id.view_progress);
int viewWidth = viewProgress.getWidth();
TranslateAnimation move = new TranslateAnimation(-(getScreenWidth() / 2) + viewWidth / 2, (getScreenWidth() / 2) + viewWidth / 2 + viewWidth, 0, 0);
move.setDuration(1000);
TranslateAnimation move1 = new TranslateAnimation(-viewWidth, 0, 0, 0);
move1.setDuration(500);
ScaleAnimation laftOut = new ScaleAnimation(0, 1, 1, 1);
laftOut.setDuration(500);
animationSet = new AnimationSet(true);
animationSet.addAnimation(move);
animationSet.addAnimation(move1);
animationSet.addAnimation(laftOut);
animationSet.addAnimation(AnimationUtils.loadAnimation(getApplicationContext(), R.anim.slideout));
startAnimation();
}
private void startAnimation() {
viewProgress.startAnimation(animationSet);
new android.os.Handler().postDelayed(new Runnable() {
@Override
public void run() {
startAnimation();
}
}, 1000);
}
public static int getScreenWidth() {
return Resources.getSystem().getDisplayMetrics().widthPixels;
}
}
8. Now run and test your app.(Tested with API 23).
Very Nice
ReplyDeleteNice
ReplyDeleteThanks for this, keep sharing
ReplyDeletethanks
ReplyDeleteStill Works with API 28 :)
ReplyDeleteGreat post.
ReplyDeletehttps://www.sandiegoreader.com/users/ChristopherWaterman/
This Is Really Useful And Nice Information
ReplyDeletehttps://forum.freeadvice.com/members/davidparker.710638/