Colorful ListView Template
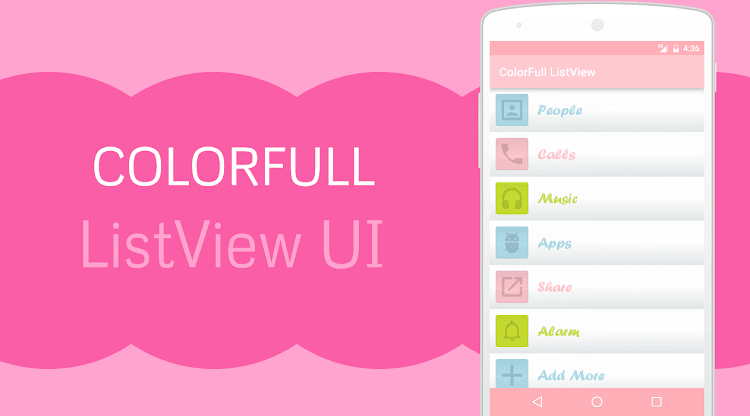
Android ListView is the great tool to show items in list because it reduces the cache level of application. Designing ListView User Interface is the hardest part for some developers. It is better if ListView templates available in on-line.
Here I Shared my ListView UI. In my ListView UI I used different color TextView in different list Items. I also used custom font for TextView. In this ListView the Icons not in color. The Icons get color at the run time. I tinted the icons in MULTIPLY mode. Icons are gray colored placed in drawable directory. I used gradient color for the TextView present in the ListView. And the ListView background is the gray gradient and the gradient level is reduced at the time it get click.
This UI is looks like designed for baby. I attache the PSD file in this project for use your custom icon. Let’s start with code. 1.Create now android project with empty activity.
2.Create new xml Drawables.
list_gradient_normal.xml<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<gradient
android:angle="90"
android:startColor="#e3e7e8"
android:centerColor="#fdfdfd"
android:endColor="#fdfdfd" />
</shape>
list_gradient_pressed.xml<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<gradient
android:angle="90"
android:endColor="#fdfdfd"
android:centerColor="#fdfdfd"
android:startColor="#f5f8f9" />
</shape>
list_gradient.xml<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true" android:drawable="@drawable/list_gradient_pressed" />
<item android:drawable="@drawable/list_gradient_normal" />
</selector>
4.Create new layout file for single list item.
list_item.xml<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="80dp"
android:background="@drawable/list_gradient"
android:gravity="center"
android:orientation="horizontal">
<ImageView
android:id="@+id/likes_list_image"
android:layout_width="55dp"
android:layout_height="55dp"
android:layout_margin="10dp"
android:src="@drawable/icon_add"
android:tint="@color/list_color_2a"
android:tintMode="multiply" />
<TextView
android:id="@+id/likes_list_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:text="Add more"
android:textSize="25dp" />
</LinearLayout>
5.Add ListView wiget in activity_main.xml.activity_main.xml<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ListView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/stock_list" />
</RelativeLayout>
6.Add colors to colors.xml file in Values directory.colors.xml<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#ffcacf</color>
<color name="colorPrimaryDark">#ffafb7</color>
<color name="colorAccent">#FF4081</color>
<color name="list_color_1">#b3e1ee</color>
<color name="list_color_1a">#6ac6dd</color>
<color name="list_color_2">#ffcacf</color>
<color name="list_color_2a">#fea1ac</color>
<color name="list_color_3">#cde431</color>
<color name="list_color_3a">#a8ca02</color>
</resources>
7.If you want change color of StatusBar, ActionBar and NavigationBar Add these values to styles.xml.styles.xml<resources xmlns:tools="http://schemas.android.com/tools">
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="android:navigationBarColor" tools:targetApi="21">@color/colorPrimaryDark</item>
</style>
</resources>
8. Create assets directory and paste the forte.ttf file.forte.ttf9. Create New class AppController.java We use this class for improve the performance of font applying.
AppController.javapackage com.androidramp.colorfulllistview;
import android.app.Application;
import android.graphics.Typeface;
import android.widget.TextView;
public class AppController extends Application {
public static Typeface font;
public static String forte = "forte.ttf";
@Override
public void onCreate() {
super.onCreate();
font = Typeface.createFromAsset(getAssets(), forte);
}
public static void Forte(TextView textView) {
textView.setTypeface(AppController.font);
}
}
10.Add class Appcontroller as name of AndroidManifest.xmlAndroidManifest.xml<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.androidramp.colorfulllistview" >
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:name=".AppController"
android:theme="@style/AppTheme" >
<activity android:name=".MainActivity" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
11. Create new class List.java.List.javapackage com.androidramp.colorfulllistview;
public class List {
private int imageId;
private String name;
public List() {
// TODO Auto-generated constructor stub
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void setImageId(int id) {
this.imageId = id;
}
public int getImageId() {
return imageId;
}
}
12. Create new class ListAdapter.java.ListAdapter.javapackage com.androidramp.colorfulllistview;
import android.content.Context;
import android.graphics.LinearGradient;
import android.graphics.Shader;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import java.util.ArrayList;
public class ListAdapter extends ArrayAdapter {
ArrayList arrayList;
LayoutInflater vi;
int Resource;
ViewHolder holder;
Context cc;
public ListAdapter(Context context, int resource, ArrayList objects) {
super(context, resource, objects);
cc = context;
vi = (LayoutInflater) context
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
Resource = resource;
arrayList = objects;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
View v = convertView;
if (v == null) {
holder = new ViewHolder();
v = vi.inflate(Resource, null);
holder.tvName = (TextView) v.findViewById(R.id.likes_list_name);
holder.ivImage = (ImageView) v.findViewById(R.id.likes_list_image);
v.setTag(holder);
} else {
holder = (ViewHolder) v.getTag();
}
holder.tvName.setText(arrayList.get(position).getName());
holder.ivImage.setImageDrawable(cc.getResources().getDrawable(arrayList.get(position).getImageId()));
AppController.Forte(holder.tvName);
Color(holder.tvName, position);
return v;
}
static class ViewHolder {
public TextView tvName;
public ImageView ivImage;
}
private void Color(TextView tv, int position) {
int mod = position % 3;
switch (mod) {
case 0:
GradientColor(tv, GetColor(R.color.list_color_1), GetColor(R.color.list_color_1a));
holder.ivImage.setColorFilter(cc.getResources().getColor(R.color.list_color_1), android.graphics.PorterDuff.Mode.MULTIPLY);
break;
case 1:
GradientColor(tv, GetColor(R.color.list_color_2), GetColor(R.color.list_color_2a));
holder.ivImage.setColorFilter(cc.getResources().getColor(R.color.list_color_2), android.graphics.PorterDuff.Mode.MULTIPLY);
break;
case 2:
GradientColor(tv, GetColor(R.color.list_color_3), GetColor(R.color.list_color_3a));
holder.ivImage.setColorFilter(cc.getResources().getColor(R.color.list_color_3), android.graphics.PorterDuff.Mode.MULTIPLY);
break;
default:ffff
break;
}
}
private void GradientColor(TextView tv, int color1, int color2) {
Shader shader = new LinearGradient(
0, 0, 0, tv.getTextSize(),
color1, color2, Shader.TileMode.CLAMP);
tv.getPaint().setShader(shader);
}
private int GetColor(int id) {
return cc.getResources().getColor(id);
}
}
13. And this goes to your MainActivity.MainActivity.javapackage com.androidramp.colorfulllistview;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.widget.ListView;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
ArrayList arrayList;
ListAdapter adapter;
String[] title = new String[]{
"People",
"Calls",
"Music",
"Apps",
"Share",
"Alarm",
"Add More",
};
int[] imageId = new int[]{
R.drawable.icon_people,
R.drawable.icon_calls,
R.drawable.icon_musiq,
R.drawable.icon_apps,
R.drawable.icon_share,
R.drawable.icon_alarm,
R.drawable.icon_add,
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// custom list
ListView listView = (ListView) findViewById(R.id.stock_list);
arrayList = new ArrayList<>();
adapter = new ListAdapter(getApplicationContext(), R.layout.list_item, arrayList);
listView.setDividerHeight(1);
listView.setAdapter(adapter);
for (int i = 0; i < title.length; i++) {
List adpacksList = new List();
adpacksList.setImageId(imageId[i]);
adpacksList.setName(title[i]);
arrayList.add(adpacksList);
}
adapter.notifyDataSetChanged();
}
}
Now run and test your project.
This is a wonderful article, Given so much info in it, These type of articles keeps the users interest in the website, and keep on sharing more ... good luck.
ReplyDeleteAndroid Training in velachery | Android Training in chennai | Android Training in chennai with placement
Thank you for sharing such wonderful information
ReplyDeletehttps://forum.freeadvice.com/members/davidparker.710638/
https://bayanlarsitesi.com/
ReplyDeleteGöktürk
Yenidoğan
Şemsipaşa
Çağlayan
H6XSPC
3A993
ReplyDeleteTekirdağ Boya Ustası
Tunceli Evden Eve Nakliyat
Zonguldak Evden Eve Nakliyat
Sinop Evden Eve Nakliyat
Kütahya Evden Eve Nakliyat
5AE85
ReplyDeletegörüntülü canlı sohbet
elazığ sohbet muhabbet
parasız görüntülü sohbet
telefonda görüntülü sohbet
çankırı canlı görüntülü sohbet odaları
telefonda rastgele sohbet
kadınlarla rastgele sohbet
çanakkale bedava sohbet
trabzon mobil sohbet odaları
B7AD2
ReplyDeletemuş rastgele canlı sohbet
manisa ucretsiz sohbet
çorum yabancı görüntülü sohbet siteleri
hatay en iyi görüntülü sohbet uygulamaları
kadınlarla ücretsiz sohbet
Burdur Sesli Sohbet Uygulamaları
kayseri bedava sohbet chat odaları
aksaray mobil sohbet sitesi
Ankara Canlı Sohbet Siteleri Ücretsiz
48794
ReplyDeletekarabük kadınlarla rastgele sohbet
Sakarya Görüntülü Sohbet Siteleri Ücretsiz
sakarya görüntülü canlı sohbet
Adana Telefonda Rastgele Sohbet
hatay ücretsiz görüntülü sohbet uygulamaları
Batman En İyi Ücretsiz Sohbet Uygulamaları
ordu parasız sohbet siteleri
uşak canlı sohbet
muğla canlı sohbet uygulamaları